ONE STOP FOR ALL STUDY MATERIALS & LAB PROGRAMS
Click on MENU to Browse between Subjects...
ADVANCED JAVA AND J2EE
[As per Choice Based Credit System (CBCS) scheme]
(Effective from the academic year 2017 -2018)
SEMESTER - V
Subject Code 17CS553
IA Marks 40
Number of Lecture Hours/Week 03
Exam Marks 60
Advertisement
CLICK ON THE QUESTIONS TO VIEW ANSWER
These Questions are being framed for helping the students in the "FINAL Exams" Only
(Remember for Internals the Question Paper is set by your respective teachers).
Questions may be repeated, just to show students how VTU can frame Questions.
- ADMIN
Mobile User: Use the Mobile on LANDSCAPE MODE to better view of Images
Beginning with JDK 5, Java added two important features: Autoboxing and auto-unboxing.
Autoboxing is the process by which a primitive type is automatically encapsulated (boxed) into its equivalent type wrapper whenever an object of that type is needed. There is no need to explicitly construct an object.
Auto-unboxing is the process by which the value of a boxed object is automatically extracted (unboxed) from a type wrapper when its value is needed. There is no need to call a method such as intValue( ) or doubleValue( ).
With Autoboxing it is no longer necessary to manually construct an object in order to wrap a primitive type. You need only assign that value to a type-wrapper reference. Java automatically constructs the object for you. For example, here is the modern way to construct an Integer object that has the value 100:
Integer iOb = 100; // autobox an int
Notice that no object is explicitly created through the use of new. Java handles this for you, automatically.
To unbox an object, simply assign that object reference to a primitive-type variable.
For example, to unbox iOb, you can use this line:
int i = iOb; // auto-unbox.
Java handles the details for you.
Here is the preceding program rewritten to use Autoboxing/unboxing:
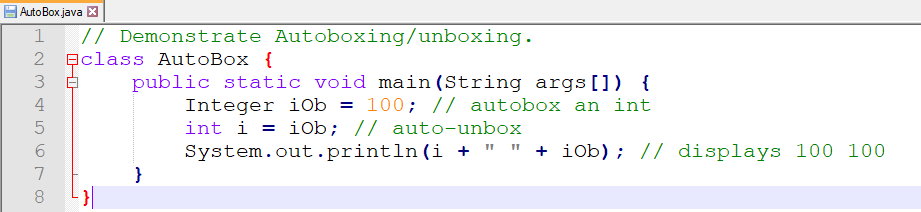
Fig 1.1: Demonstration of Auto-Boxing and Auto-Unboxing.
Java provides type wrappers, which are classes that encapsulate a primitive type within an object.
The type wrappers are Double, Float, Long, Integer, Short, Byte, Character, and Boolean. These classes offer a wide array of methods that allow you to fully integrate the primitive types into Java's object hierarchy. Each is briefly examined next.
2.1 Character
Character is a wrapper around a char. The constructor for Character is
Character(char ch)
Here, ch specifies the character that will be wrapped by the Character object being created. To obtain the char value contained in a Character object, call charValue( ), shown here:
char charValue( )
It returns the encapsulated character.
2.2 The Numeric Type Wrappers
By far, the most commonly used type wrappers are those that represent numeric values. These are Byte, Short, Integer, Long, Float, and Double. All of the numeric type wrappers inherit the abstract class Number. Number declares methods that return the value of an object in each of the different number formats. These methods are shown here:
byte byteValue( )
double doubleValue( )
float floatValue( )
int intValue( )
long longValue( )
short shortValue( )
For example, doubleValue( ) returns the value of an object as a double, floatValue( ) returns the value as a float, and so on. These methods are implemented by each of the numeric type wrappers.
All of the numeric type wrappers define constructors that allow an object to be constructed from a given value, or a string representation of that value. For example, here are the constructors defined for Integer:
Integer(int num)
Integer(String str)
If str does not contain a valid numeric value, then a NumberFormatException is thrown.
All of the type wrappers override toString( ). It returns the human-readable form of the value contained within the wrapper. This allows you to output the value by passing a type wrapper object to println( ), for example, without having to convert it into its primitive type.
The following program demonstrates how to use a numeric type wrapper to encapsulate a value and then extract that value.
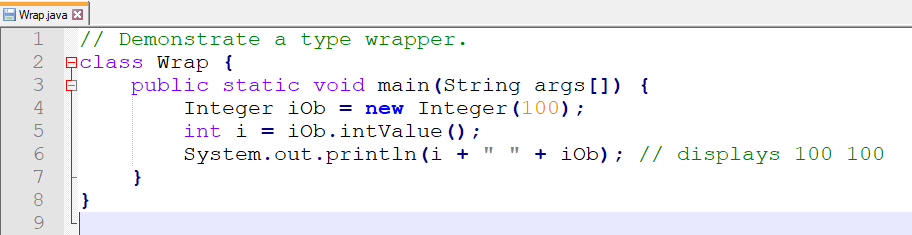
Fig 2.1: Demonstration of numeric type wrapper .
This program wraps the integer value 100 inside an Integer object called iOb. The program then obtains this value by calling intValue( ) and stores the result in i.
The process of encapsulating a value within an object is called boxing. Thus, in the program, this line boxes the value 100 into an Integer:
Integer iOb = new Integer(100); //boxing
The process of extracting a value from a type wrapper is called unboxing. For example, the program unboxes the value in iOb with this statement:
int i = iOb.intValue(); //unboxing
Although you can't inherit a superclass when declaring an enum, all enumerations automatically inherit one: java.lang.Enum. This class defines several methods that are available for use by all enumerations.
i. Ordinal( ):
We can obtain a value that indicates an enumeration constant's position in the list of constants. This is called its ordinal value, and it is retrieved by calling the ordinal( ) method, shown here:
final int ordinal( )
It returns the ordinal value of the invoking constant. Ordinal values begin at zero. Thus, in the Apple enumeration, Jonathan has an ordinal value of zero, GoldenDel has an ordinal value of 1, RedDel has an ordinal value of 2, and so on.
ii. compareTo( ):
You can compare the ordinal value of two constants of the same enumeration by using the compareTo( ) method. It has this general form:
final int compareTo(enum-type e)
Here, enum-type is the type of the enumeration, and e is the constant being compared to the invoking constant. Remember, both the invoking constant and e must be of the same enumeration. If the invoking constant has an ordinal value less than e's, then compareTo( ) returns a negative value. If the two ordinal values are the same, then zero is returned. If the invoking constant has an ordinal value greater than e's, then a positive value is returned.
iii. equals( ):
You can compare for equality an enumeration constant with any other object by using equals( ), which overrides the equals( ) method defined by Object. Although equals( ) can compare an enumeration constant to any other object, those two objects will only be equal if they both refer to the same constant, within the same enumeration. Simply having ordinal values in common will not cause equals( ) to return true if the two constants are from different enumerations.
Remember, you can compare two enumeration references for equality by using = =. The following program demonstrates the ordinal( ), compareTo( ), and equals( ) methods:
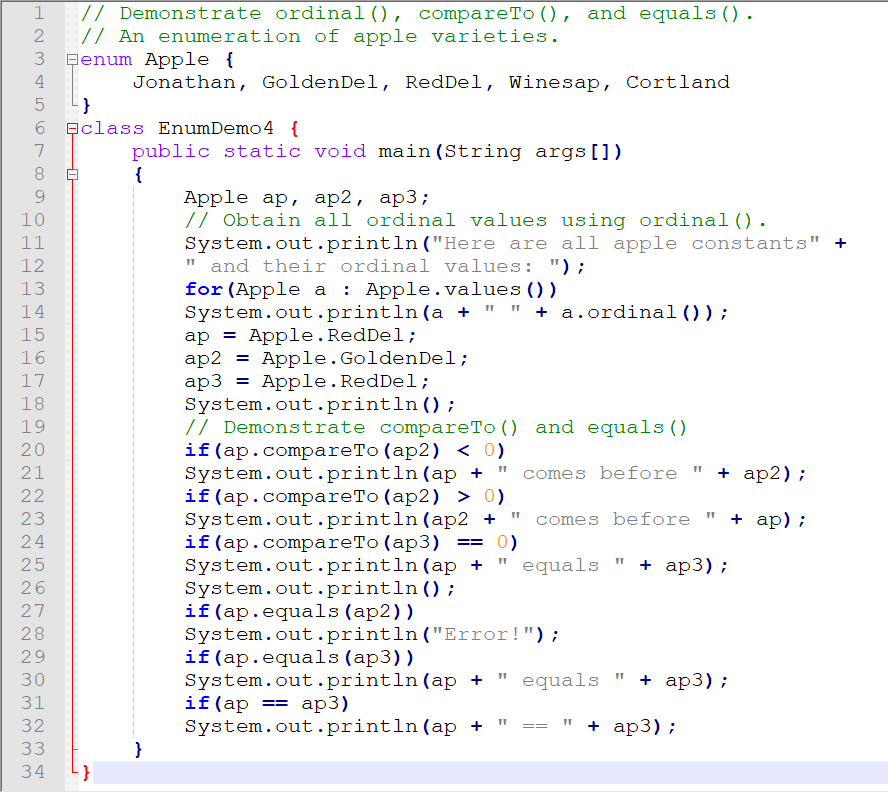
Fig 3.1: Demonstrations of ordinal( ), compareTo( ), and equals( ) methods.
A single-member annotation contains only one member. It works like a normal annotation except that it allows a shorthand form of specifying the value of the member. When only one member is present, you can simply specify the value for that member when the annotation is applied—you don't need to specify the name of the member. However, in order to use this shorthand, the name of the member must be value.
Here is an example that creates and uses a single-member annotation:
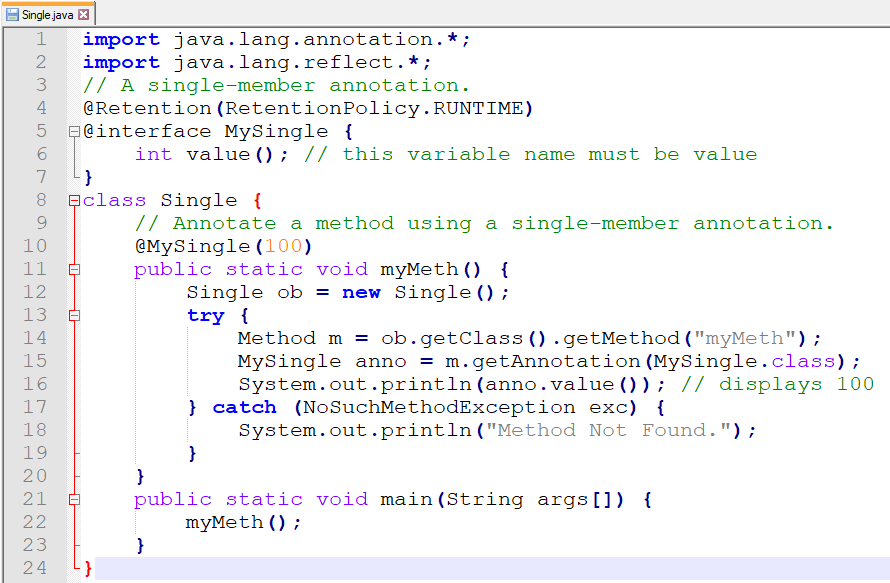
Fig 4.1: Demonstrations to creates and uses a single-member annotation
Beginning with JDK 5, a new facility was added to Java that enables you to embed supplemental information into a source file. This information, called an annotation, does not change the actions of a program. Thus, an annotation leaves the semantics of a program unchanged. However, this information can be used by various tools during both development and deployment. For example, an annotation might be processed by a source-code generator. The term metadata is also used to refer to this feature, but the term annotation is the most descriptive and more commonly used.
5.1 The Built-In Annotations
Java defines many built-in annotations. Most are specialized, but seven are general purpose. Of these, four are imported from java.lang.annotation: @Retention, @Documented, @Target, and @Inherited. Three - @Override, @Deprecated, and @SuppressWarnings - are included in java.lang. Each is described here.
5.1.1 @Retention
@Retention is designed to be used only as an annotation to another annotation. It specifies the retention policy as described earlier in this chapter.
5.1.2 @Documented
The @Documented annotation is a marker interface that tells a tool that an annotation is to be documented. It is designed to be used only as an annotation to an annotation declaration.
5.1.3 @Target
The @Target annotation specifies the types of declarations to which an annotation can be applied. It is designed to be used only as an annotation to another annotation. @Target takes one argument, which must be a constant from the ElementType enumeration. This argument specifies the types of declarations to which the annotation can be applied. The constants are shown here along with the type of declaration to which they correspond.
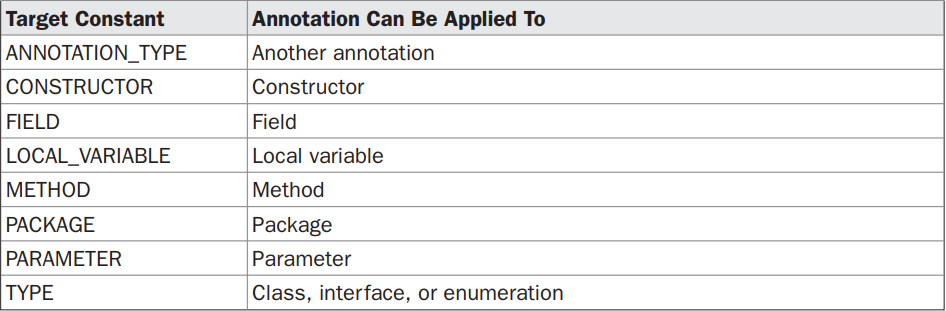
Fig 5.1: @Target Constants with Declaration
You can specify one or more of these values in a @Target annotation. To specify multiple values, you must specify them within a braces-delimited list. For example, to specify that an annotation applies only to fields and local variables, you can use this @Target annotation:
@Target( { ElementType.FIELD, ElementType.LOCAL_VARIABLE } )
5.1.4 @Inherited
@Inherited is a marker annotation that can be used only on another annotation declaration. Furthermore, it affects only annotations that will be used on class declarations. @Inherited causes the annotation for a superclass to be inherited by a subclass. Therefore, when a request for a specific annotation is made to the subclass, if that annotation is not present in the subclass, then its superclass is checked. If that annotation is present in the superclass, and if it is annotated with @Inherited, then that annotation will be returned.
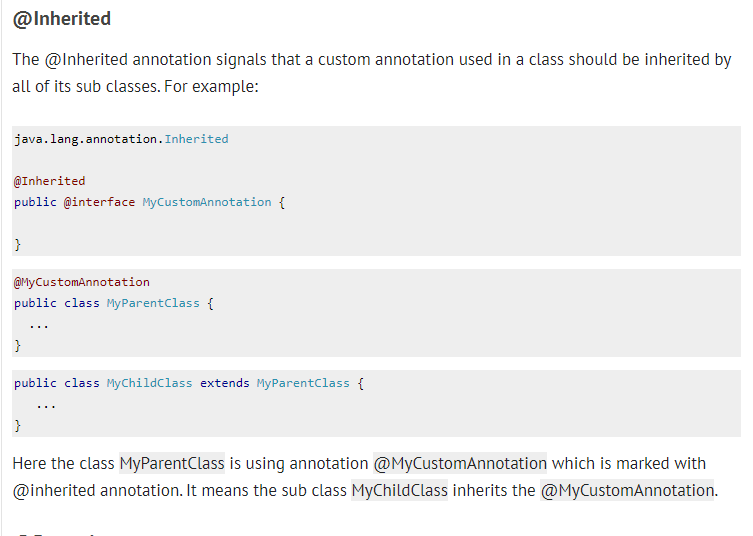
Fig 5.2: Demonstration of @Inherited Marker Annotation
5.1.5 @Override
@Override is a marker annotation that can be used only on methods. A method annotated with @Override must override a method from a superclass. If it doesn't, a compile-timeerror will result. It is used to ensure that a superclass method is actually overridden, and not simply overloaded.
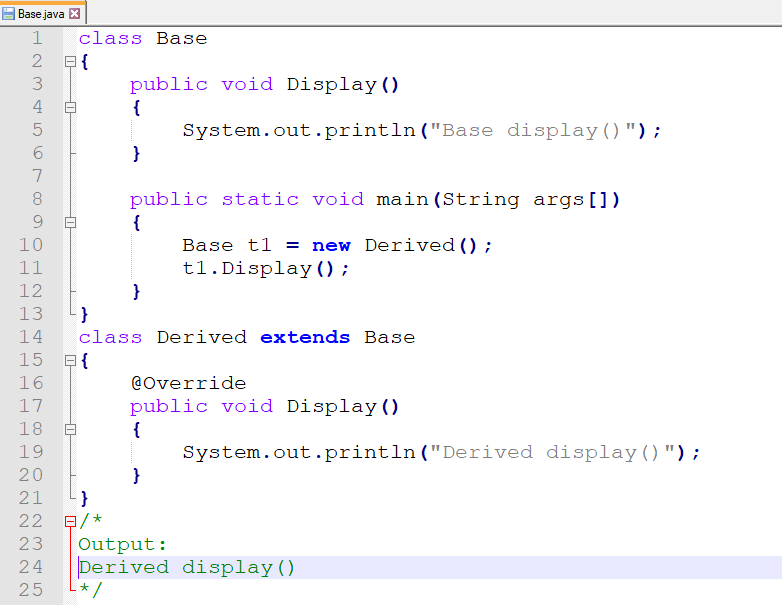
Fig 5.3: Demonstration of @Override Marker Annotation
5.1.6 @Deprecated
@Deprecated is a marker annotation. It indicates that a declaration is obsolete and has been replaced by a newer form.
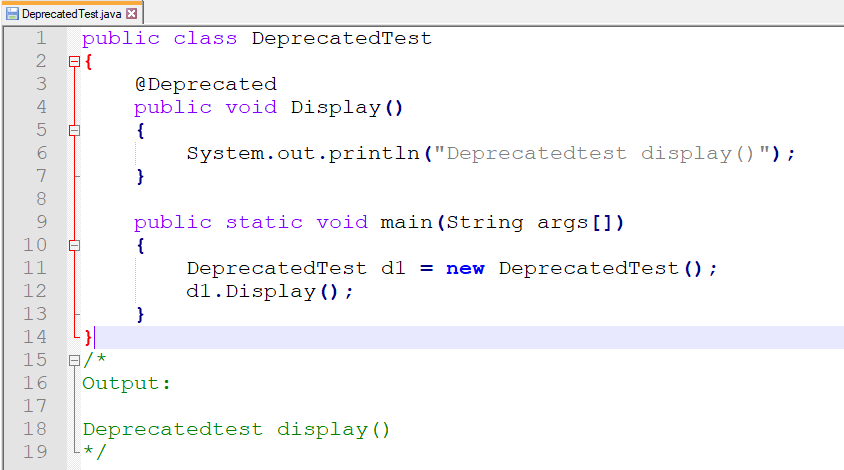
Fig 5.4: Demonstration of @Deprecated Marker Annotation
5.1.7 @SuppressWarnings
@SuppressWarnings specifies that one or more warnings that might be issued by the compiler are to be suppressed. The warnings to suppress are specified by name, in string form. This annotation can be applied to any type of declaration.
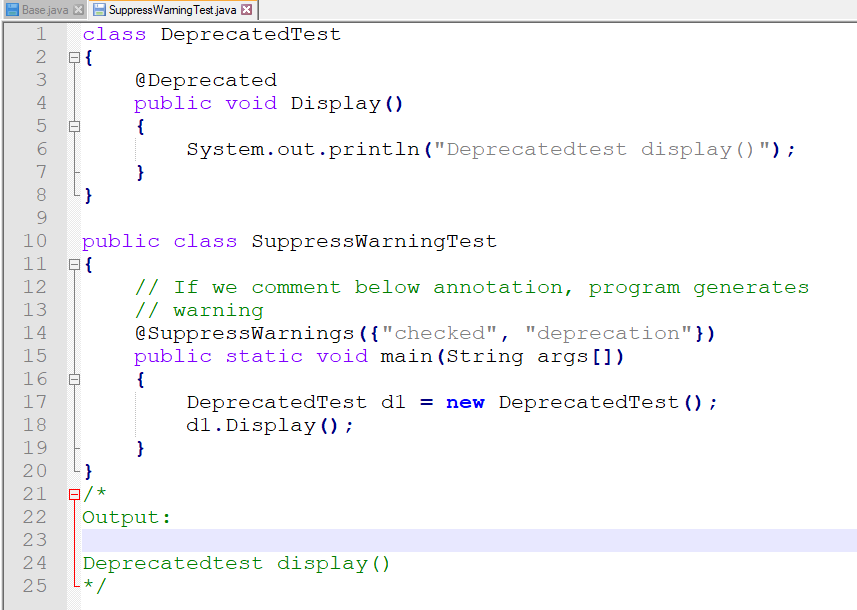
Fig 5.5: Demonstration of @SuppressWarnings Marker Annotation
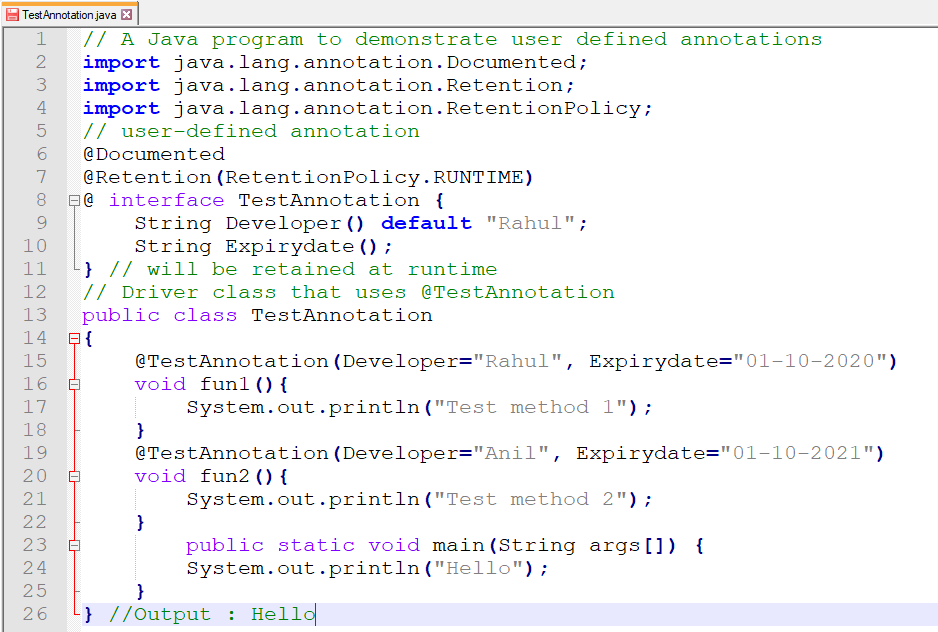
Fig 5.6: Demonstration of User Defined Annotation