NOTE!
Click on MENU to Browse between Subjects...17CS553 - ADVANCED JAVA AND J2EE
Answer Script for Module 2
Solved Previous Year Question Paper
CBCS SCHEME
ADVANCED JAVA AND J2EE
[As per Choice Based Credit System (CBCS) scheme]
(Effective from the academic year 2019 -2020)
SEMESTER - V
Subject Code 17CS553
IA Marks 40
Number of Lecture Hours/Week 03
Exam Marks 60
These Questions are being framed for helping the students in the "FINAL Exams" Only
(Remember for Internals the Question Paper is set by your respective teachers).
Questions may be repeated, just to show students how VTU can frame Questions.
- ADMIN
The
ArrayList
class extends
AbstractList
and implements the
List
interface.
ArrayList
is a generic class that has this declaration: class ArrayList<E>
Here,
E
specifies the type of objects that the list will hold.
ArrayList
supports dynamic arrays that can grow as needed. In Java, standard arrays
are of a fixed length. After arrays are created, they cannot grow or shrink,
which means that you must know in advance how many elements an array will
hold. But, sometimes, you may not know until run time precisely how large an
array you need. To handle this situation, The Collections Framework defines
ArrayList
. In essence, an
ArrayList
is a variable-length array of object references.
That is, an
ArrayList
can dynamically increase or decrease in size. Array lists are created with
an initial size. When this size is exceeded, the collection is automatically
enlarged. When objects are removed, the array can be shrunk.
ArrayList
has the constructors shown here:
ArrayList( )
ArrayList(Collection<? extends E> c)
ArrayList(int capacity)
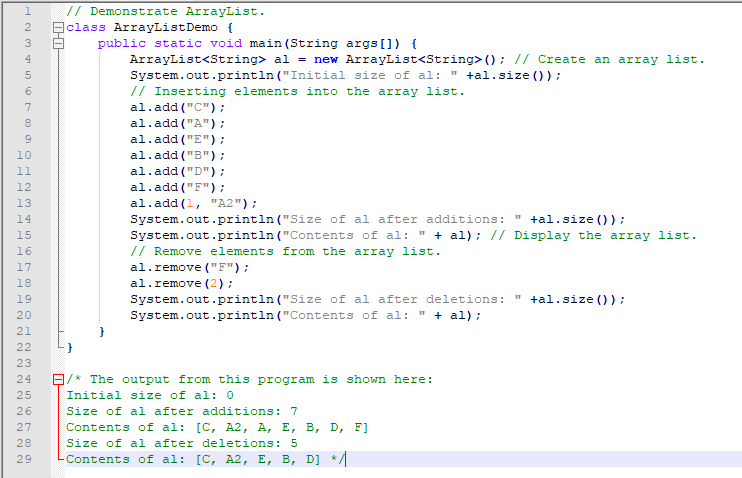
The
Queue
interface extends
Collection
and declares the behavior of a queue, which is often a first-in, first-out
list. However, there are types of queues in which the ordering is based upon
other criteria.
Queue
is a generic interface that has this declaration: interface Queue<E>
Here,
E
specifies the type of objects that the queue will hold. The methods defined
by Queue
Different Methods
E element()
Returns the element at the head of the queue. The element is not removed.
It throws
NoSuchElementException
if the queue is empty.
boolean offer(E obj)
Attempts to add obj to the queue. Returns true if obj was added and false
otherwise.
E peek()
Returns the element at the head of the queue. It returns null if the queue
is empty. The element is not removed.
E poll()
Returns the element at the head of the queue, removing the element in the
process. It returns null if the queue is empty.
E remove()
Removes the element at the head of the queue, returning the element in the
process. It throws
NoSuchElementException
if the queue is empty.
Several methods throw a
ClassCastException
when an object is incompatible with the elements in the queue. A
NullPointerException
is thrown if an attempt is made to store a
null
object and
null
elements are not allowed in the queue. An
IllegalArgumentException
is thrown if an invalid argument is used. An
IllegalStateException
is thrown if an attempt is made to add an element to a fixed-length queue
that is full. A
NoSuchElementException
is thrown if an attempt is made to remove an element from an empty queue.
Despite its simplicity,
Queue
offers several points of interest. First, elements can only be removed from
the head of the queue. Second, there are two methods that obtain and remove
elements:
poll()
and
remove()
. The difference between them is that
poll()
returns
null
if the queue is empty, but
remove()
throws an exception. Third, there are two methods,
element()
and
peek( )
, that obtain but don’t remove the element at the head of the queue.
They differ only in that
element()
throws an exception if the queue is empty, but
peek( )
returns
null
. Finally, notice that
offer()
only attempts to add an element to a queue. Because some queues have a fixed
length and might be full,
offer()
can fail.
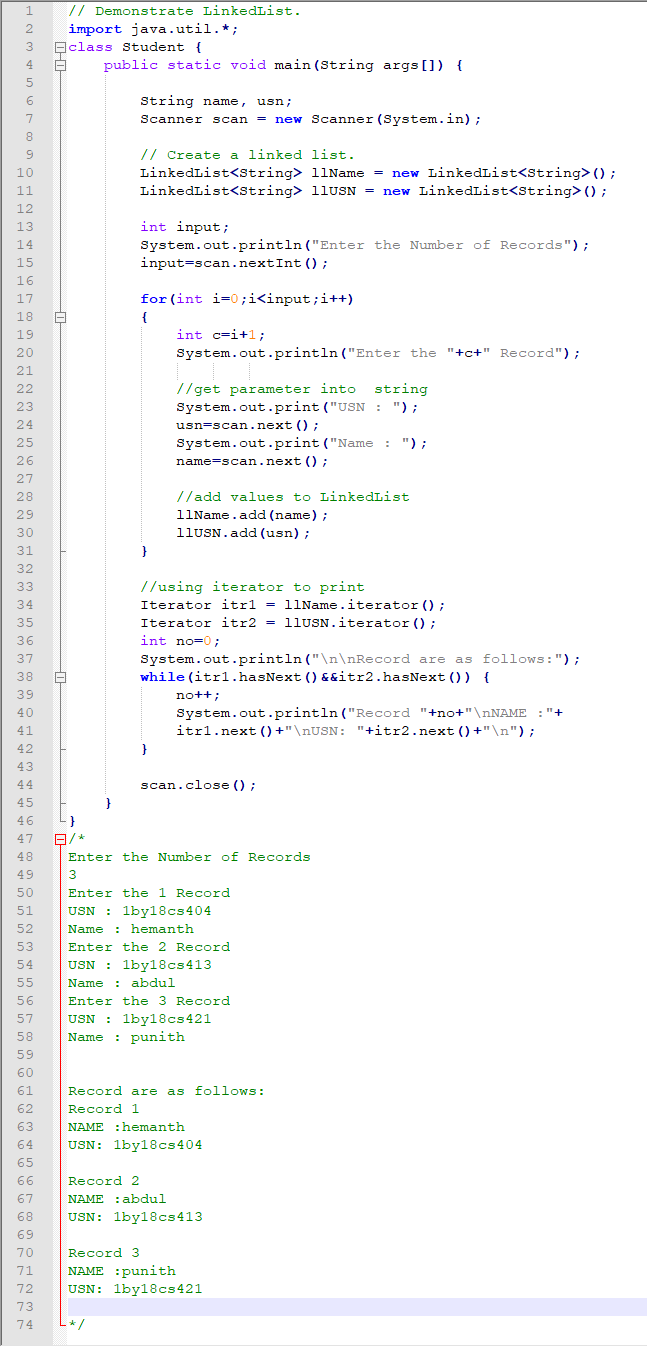
ArrayList
supports dynamic arrays that can grow as needed. In Java, standard arrays
are of a fixed length. After arrays are created, they cannot grow or shrink,
which means that you must know in advance how many elements an array will
hold. But, sometimes, you may not know until run time precisely how large an
array you need.
binarySearch()
The
binarySearch( )
method uses a binary search to find a specified value. This method must be
applied to sorted arrays.
Here are some of its forms.
static int binarySearch(char
array
[ ], char
value
)
static int binarySearch(double
array
[ ], double
value
)
static int binarySearch(float
array
[ ], float
value
)
static int binarySearch(long
array
[ ], long
value
)
static int binarySearch(short
array
[ ], short
value
)
static int binarySearch(Object
array
[ ], Object
value
)
static <T> int binarySearch(T[ ]
array
, T
value
, Comparator<? super T>
c
)
Here,
array
is the array to be searched, and
value
is the value to be located. The last two forms throw a
ClassCastException
if
array
contains elements that cannot be compared (for example,
Double
and
StringBuffer
) or if
value
is not compatible with the types in
array.
In the last form, the
Comparator
c
is used to determine the order of the elements in
array.
In all cases, if
value
exists in
array,
the index of the element is returned. Otherwise, a negative value is
returned.
copyOf()
The
copyOf( )
method was added by Java SE 6. It returns a copy of an array and has the
following forms:
static boolean[ ] copyOf(boolean[ ]
source
, int
len
)
static byte[ ] copyOf(byte[ ]
source
, int
len
)
static char[ ] copyOf(char[ ]
source
, int
len
)
static double[ ] copyOf(double[ ]
source
, int
len
)
static <T> T[ ] copyOf(T[ ]
source
, int
len
)
static <T,U> T[ ] copyOf(U[ ]
source
, int
len
, Class<? extends T[ ]>
resultT
)
The original array is specified by
source,
and the length of the copy is specified by
len
. If the copy is longer than
source
, then the copy is padded with zeros (for numeric arrays),
null
s (for object arrays), or
false
(for boolean arrays). If the copy is shorter than
source
, then the copy is truncated. In the last form, the type of
resultT
becomes the type of the array returned. If
len
is negative, a
NegativeArraySizeException
is thrown. If
source
is
null
, a
NullPointerException
is thrown. If
resultT
is incompatible with the type of
source,
an
ArrayStoreException
is thrown.
equals()
The
equals( )
method returns
true
if two arrays are equivalent. Otherwise, it returns
false
. The
equals( )
method has the following forms:
static boolean equals(boolean
array1
[ ], boolean
array2
[ ])
static boolean equals(byte
array1
[ ], byte
array2
[ ])
static boolean equals(char
array1
[ ], char
array2
[ ])
static boolean equals(double
array1
[ ], double
array2
[ ])
static boolean equals(long
array1
[ ], long
array2
[ ])
static boolean equals(short
array1
[ ], short
array2
[ ])
static boolean equals(Object
array1
[ ], Object
array2
[ ])
Here,
array1
and
array2
are the two arrays that are compared for equality.
fill()
The
fill( )
method assigns a value to all elements in an array. In other words, it fills
an
array with a specified value. The
fill( )
method has two versions. The first version, which
has the following forms, fills an entire array:
static void fill(boolean
array
[ ], boolean
value
)
static void fill(double
array
[ ], double
value
)
static void fill(float
array
[ ], float
value
)
static void fill(int
array
[ ], int
value
)
static void fill(long
array
[ ], long
value
)
static void fill(short
array
[ ], short
value
)
static void fill(Object
array
[ ], Object
value
)
Here,
value
is assigned to all elements in
array.
Set Interface
The
Set
interface defines a set. It extends
Collection
and declares the behavior of a collection that does not allow duplicate
elements. Therefore, the
add( )
method returns
false
if an attempt is made to add duplicate elements to a set. It does not define
any additional methods of its own.
Set
is a generic interface that has this declaration: interface Set<E>
Here,
E
specifies the type of objects that the set will hold.
List Interface
The
List
interface extends
Collection
and declares the behavior of a collection that stores a sequence of
elements. Elements can be inserted or accessed by their position in the
list, using a zero-based index. A list may contain duplicate elements.
List
is a generic interface that has this declaration: interface List<E>
Here,
E
specifies the type of objects that the list will hold.
In addition to the methods defined by
Collection
,
List
defines some of its own, which are summarized in Table 17-2. Note again that
several of these methods will throw an
UnsupportedOperationException
if the list cannot be modified, and a
ClassCastException
is generated when one object is incompatible with another, such as when an
attempt is made to add an incompatible object to a list. Also, several
methods will throw an
IndexOutOfBoundsException
if an invalid index is used. A
NullPointerException
is thrown if an attempt is made to store a
null
object and
null
elements are not allowed in the list. An
IllegalArgumentException
is thrown if an invalid argument is used.
To the versions of
add()
and
addAll()
defined by
Collection
,
List
adds the methods
add(int, E)
and
addAll(int, Collection)
. These methods insert elements at the specified index. Also, the semantics
of
add(E)
and
addAll(Collection)
defined by
Collection
are changed by
List
so that they add elements to the end of the list.
To obtain the object stored at a specific location, call
get()
with the index of the object. To assign a value to an element in the list,
call
set()
, specifying the index of the object to be changed. To find the index of an
object, use
indexOf()
or
lastIndexOf()
. You can obtain a sublist of a list by calling
subList()
, specifying the beginning and ending indexes of the sublist. As you can
imagine,
subList()
makes list processing quite convenient.
Below Page NAVIGATION Links are Provided...
All the Questions on Question Bank Is SOLVED
Follow our Instagram Page:
FutureVisionBIE
https://www.instagram.com/futurevisionbie/
Message: I'm Unable to Reply to all your Emails
so, You can DM me on the Instagram Page & any other Queries.