×
NOTE!
Click on MENU to Browse between Subjects...
Advertisement
17CS664 - PYTHON APPLICATION PROGRAMMING
Answer Script for Module 4
Solved Previous Year Question Paper
CBCS SCHEME
PYTHON APPLICATION PROGRAMMING
[As per Choice Based Credit System (CBCS) scheme]
(Effective from the academic year 2017 - 2018)
SEMESTER - VI
Subject Code 17CS664
IA Marks 40
Number of Lecture Hours/Week 3
Exam Marks 60
Advertisement
These Questions are being framed for helping the students in the "FINAL Exams" Only
(Remember for Internals the Question Paper is set by your respective teachers).
Questions may be repeated, just to show students how VTU can frame Questions.
- ADMIN
×
CLICK ON THE QUESTIONS TO VIEW ANSWER
ANSWER :
1.1 Classes and Object:
Python is an object-oriented programming language, and class is a basis for any object oriented programming language. Class is a user-defined data type which binds data and functions together into single entity. Class is just a prototype (or a logical entity/blue print) which will not consume any memory. An object is an instance of a class and it has physical existence. One can create any number of objects for a class. A class can have a set of variables (also known as attributes, member variables) and member functions (also known as methods).
1.2 Programmer defined types:
A class in Python can be created using a keyword class
.
Here, we are creating an empty class without any members by just using the
keyword pass
within it.
class Point:
pass
print(Point)
The output would be -
<class '__main__.Point'>
The term __main__ indicates that the class Point is in the main scope of the current module. In other words, this class is at the top level while executing the program.
Now, a user-defined data type Point
got created, and this
can be used to create any number of objects of this class. Observe the
following statements
p=Point()
Now, a reference (for easy understanding, treat reference as a pointer) to Point
object is created and is returned. This returned
reference is assigned to the object p
. The process of
creating a new object is called as instantiation
and the
object is instance
of a class. When we print an object,
Python tells which class it belongs to and where it is stored in the
memory.
print(p)
The output would be -
<__main__.Point object at 0x003C1BF0>
The output displays the address (in hexadecimal format) of the object in the memory. It is now clear that, the object occupies the physical space, whereas the class does not.
Advertisement
ANSWER :
2.1 Introduction to Functions:
Though Python is object oriented programming languages, it is possible to
use it as functional programming. There are two types of functions viz. pure functions
and modifiers
. A pure
function takes objects as arguments and does some work without
modifying any of the original argument. On the other
hand, as the name suggests, modifier function modifies the original
argument.
In practical applications, the development of a program will follow a
technique called as prototype
and patch
.
That is, solution to a complex problem starts with simple prototype
and incrementally dealing with the complications.
2.2 Pure Function:
To understand the concept of pure functions, let us consider an example of creating a class called Time. An object of class Time contains hour, minutes and seconds as attributes. Write a function to print time in HH:MM:SS format and another function to add two time objects. Note that, adding two time objects should yield proper result and hence we need to check whether number of seconds exceeds 60, minutes exceeds 60 etc, and take appropriate action.
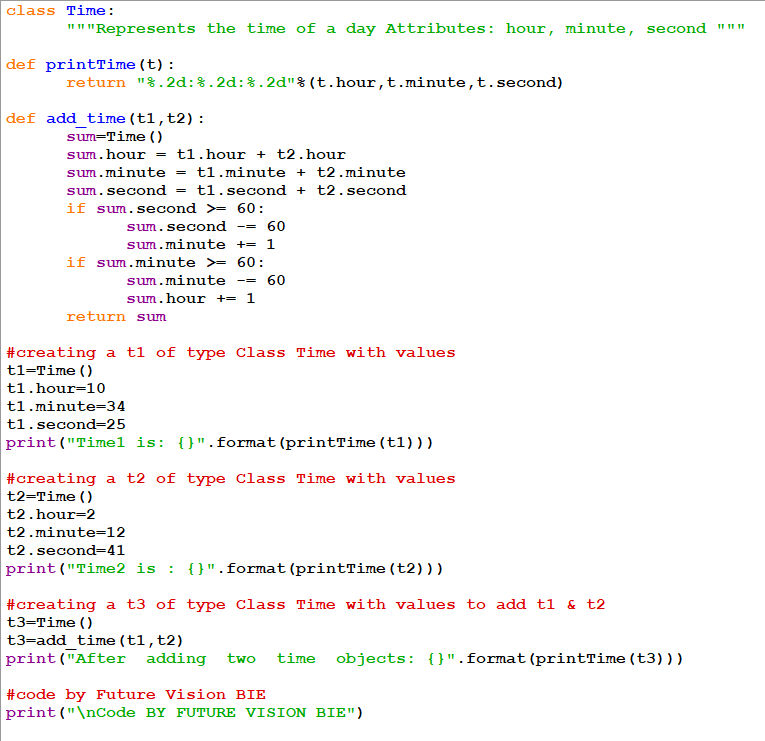
Fig 2.1 Program to demonstrate Pure Function
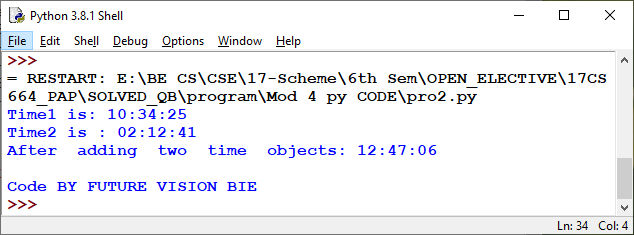
Fig 2.2 Output of Program to demonstrate Pure Function
Here, the function add_time() takes two arguments of type Time, and returns
a Time object, whereas, it is not modifying contents of its arguments t1
and t2. Such functions are called as pure functions
.
ANSWER :
3.1 Difference between Method & function:
Methods are semantically the same as functions, but there are two syntactic differences:
i. Methods are defined inside a class definition in order to make the relationship between the class and the method explicit.
ii. The syntax for invoking a method is different from the syntax for calling a function.
3.2 Working of _init_ method:
Python provides a special method called as __init__() which is similar to constructor method in other programming languages like C++/Java. The term init indicates initialization. As the name suggests, this method is invoked automatically when the object of a class is created. Consider the example given here -
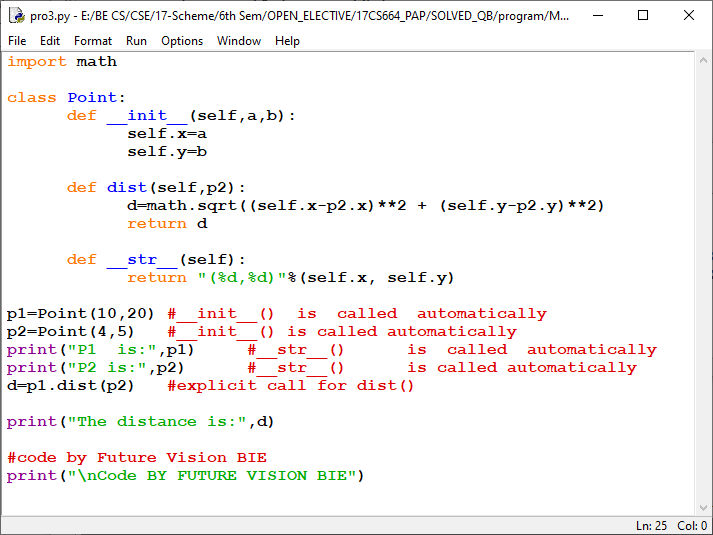
Fig 3.1 Demonstration of _init_ Method
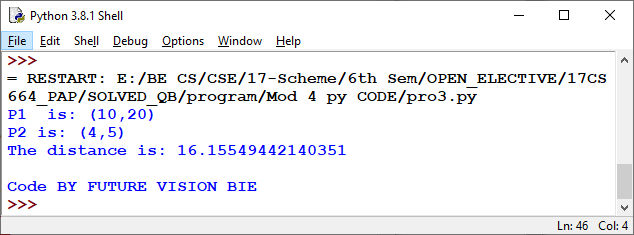
Fig 3.2 Output of Demonstration of _init_ Method
Let us understand the working of this program and the concepts involved:
Keep in mind that every method of any class must have the first argument as self
. The argument self
is a reference to
the current object. That is, it is reference to the object which invoked
the method. (Those who know C++, can relate self
with this
pointer). The object which invokes a method is also
known as subject
.
The method __init__() inside the class is an initialization method, which will be invoked automatically when the object gets created. When the statement like -
p1=Point(10,20)
is used, the __init__() method will be called automatically. The internal meaning of the above line is -
p1.__init__(10,20)
Here, p1 is the object which is invoking a method. Hence, reference to this object is created and passed to __init__() as self. The values 10 and 20 are passed to formal parameters a and b of __init__() method. Now, inside __init__() method, we have statements
self.x=10
self.y=20
This indicates, x and y are instance attributes. The value of x for the object p1 is 10 and, the value of y for the object p1 is 20.
When we create another object p2, it will have its own set of x and y. That is, memory locations of instance attributes are different for every object.
Thus, state of the object can be understood by instance attributes.
The method dist() is an ordinary member method of the class Point. As mentioned earlier, its first argument must be self. Thus, when we make a call as
d=p1.dist(p2)
a reference to the object p1 is passed as self to dist() method and p2 is passed explicitly as a second argument. Now, inside the dist()method, we are calculating distance between two point (Euclidian distance formula is used) objects. Note that, in this method, we cannot use the name p1, instead we will use self which is a reference (alias) to p1.
The next method inside the class is__str__(). It is a special method used for
string representation of user-defined object.
Usually,
print()
is used for
printing basic types in Python. But, user-defined types (class objects)
have their own meaning and a way of representation. To display such types,
we can write functions or methods like print_point() as we did in Section
4.1.2. But, more polymorphic way is to use __str__() so that, when we write
just print() in the main part of the program, the __str__() method will be
invoked automatically. Thus, when we use the statement like -
print("P1 is:",p1)
the ordinary print() method will print the portion "P1 is:" and the remaining portion is taken care by __str__() method. In fact, __str__() method will return the string format what we have given inside it, and that string will be printed by print() method.
Advertisement
ANSWER :
4.1 Attribute:
An object can contain named elements known as attributes
.
One can assign values to these attributes using dot operator. For example,
keeping coordinate points in mind, we can assign two attributes x and y for
the object p of a class Point as below -
p.x =10.0
p.y =20.0
4.2 Function returning instance value:
(This Section Cover a lot more topic than it is needed
i. Having One class as an attribute to other class
ii. Function Returning instance value
iii. Function to Calculate Centre point of A Rectangle)
It is possible to make an object of one class as an attribute to other class. To illustrate this, consider an example of creating a class called as Rectangle. A rectangle can be created using any of the following data
i. By knowing width and height of a rectangle and one corner point (ideally, a bottom-left corner) in a coordinate system
ii. By knowing two opposite corner points
Let us consider the first technique and implement the task: Write a class Rectangle containing numeric attributes width and height. This class should contain another attribute corner which is an instance of another class Point. Implement following functions -
i. A function to print corner point as an ordered-pair
ii. A function find_center() to compute center point of the rectangle
iii. A function resize() to modify the size of rectangle
The program is as given below
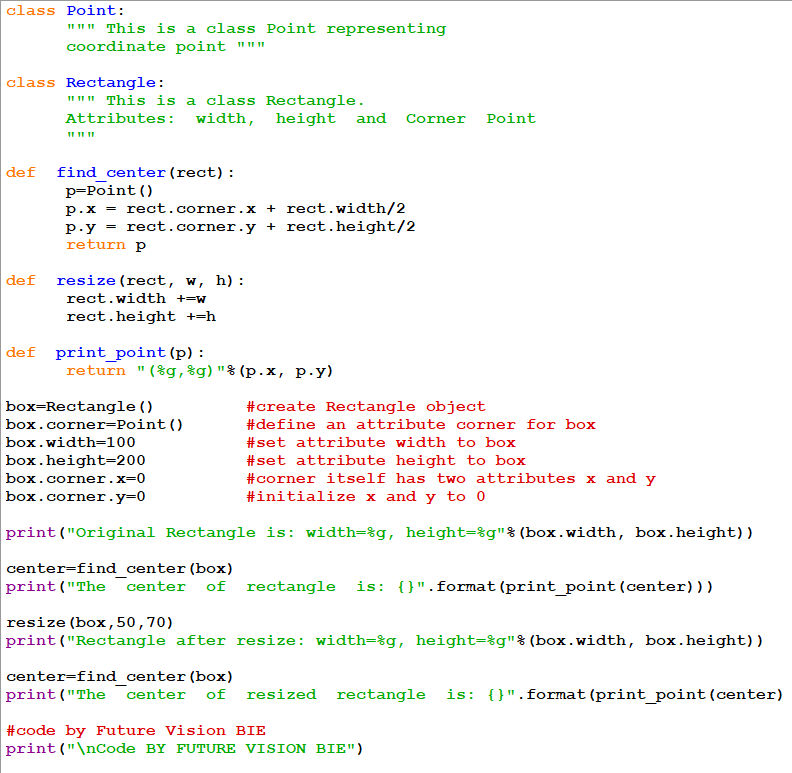
Fig 4.1 Program to Demonstrate Mentioned Functions
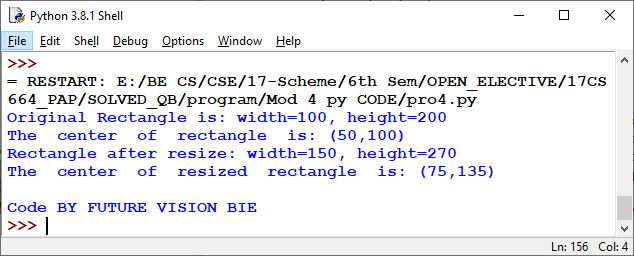
Fig 4.2 Output of Program to Demonstrate Mentioned Functions
The working of above program is explained in detail here -
Two classes Point and Rectangle have been created with suitable docstrings. As of now, they do not contain any class-level attributes.
The following statement instantiates an object of Rectangle class.
box=Rectangle()
The statement
box.corner=Point()
indicates that corner is an attribute for the object box and this attribute itself is an object of the class Point. The following statements indicate that the object box has two more attributes
box.width=100 #give any numeric value
box.width=200 #give any numeric value.
In this program, we are treating the corner point as the origin in coordinate system and hence the following assignments -
box.corner.x=0
box.corner.y=0
(Note that, instead of origin, any other location in the coordinate system can be given as corner point.)
Based on all above statements, an object diagram can be drawn as -
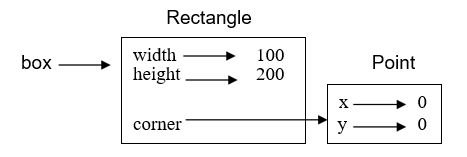
The expression box.corner.x means, "Go to the object box refers to and select the attribute named corner; then go to that object and select the attribute named x."
The function find_center() takes an object rect as an argument. So, when a call is made using the statement -
center=find_center(box)
the object rect acts as an alias for the argument box.
A local object p of type Point has been created inside this function. The attributes of p are x and y, which takes the values as the coordinates of center point of rectangle. Center of a rectangle can be computed with the help of following diagram.
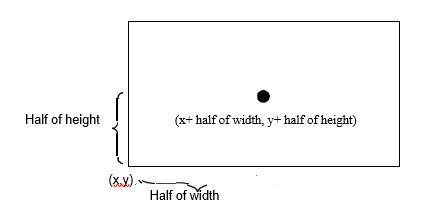
The function find_center() returns the computed center point. Note that,
the return value of a function here is an instance of some class. That is,
one can have an instance as return values
from a function.
The function resize() takes three arguments: rect - an instance of
Rectangle class and two numeric variables w and h. The values w and h are
added to existing attributes width and height. This clearly shows that objects are mutable
. State of an object can be changed by
modifying any of its attributes. When this function is called with a
statement -
resize(box,50,70)
the rect acts as an alias for box. Hence, width and height modified within the function will reflect the original object box.
Thus, the above program illustrates the concepts: object of one class is made as
attribute for object of another class, returning objects from functions
and objects are mutable.
ANSWER :
Sometimes, it is necessary to modify the underlying argument so as to
reflect the caller. That is, arguments have to be modified inside a
function and these modifications should be available to the caller. The
functions that perform such modifications are known as modifier function.
Assume that, we need to add few seconds
to a time object, and get a
new time. Then, we can write a
function as below
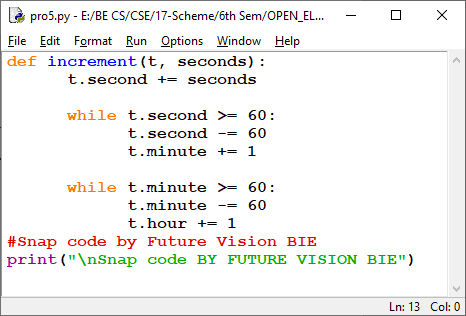
In this function, we will initially add the argument seconds to t.second.
Now, there is a chance that t.second is exceeding 60. So, we will increment
minute counter till t.second becomes lesser than 60. Similarly, till the
t.minute becomes lesser than 60, we will decrement minute counter. Note
that, the modification is done on the argument t itself. Thus, the above
function is a modifier.
Code of a Function on 2.1 Figure
×
NOTE: Each Page Provides only 5 Questions & Answer
Below Page NAVIGATION Links are Provided...
All the Questions on Question Bank Is SOLVED
Below Page NAVIGATION Links are Provided...
All the Questions on Question Bank Is SOLVED
Advertisement
lIKE OUR CONTENT SUPPORT US BY FOLLOWING US ON INSTAGRAM : @futurevisionbie
For immediate Notification Join the Telegram Channel
×
SUGGESTION: SHARE WITH ALL THE STUDENTS AND FRIENDS -ADMIN