×
NOTE!
Click on MENU to Browse between Subjects...
Advertisement
COMPUTER GRAPHICS LABORATORY WITH MINI PROJECT
[As per Choice Based Credit System (CBCS) scheme]
(Effective from the academic year 2017 - 2018)
SEMESTER - VI
Subject Code 17CSL68
IA Marks 40
Number of Lecture Hours/Week 01I + 02P
Exam Marks 60
Advertisement
17CSL68 - COMPUTER GRAPHICS LABORATORY WITH MINI PROJECT
PROGRAM - 8
Develop a menu driven program to animate a flag using Bezier Curve algorithm
Code Credits Prof Shankar R, BMSIT
DESIGN Credits Mr K B Hemanth Raj - Admin
Advertisement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 | //8. Develop a menu driven program to animate a flag using Bezier curve algorithm. #include<GL/glut.h> #include<stdio.h> #include<math.h> #define PI 3.1416 float theta = 0; struct point { GLfloat x, y, z; }; int factorial(int n) { if (n<=1) return(1); else n=n*factorial(n-1); return n; } void computeNcR(int n, int *hold_ncr_values) { int r; for(r=0; r<=n; r++) //start from nC0, then nC1, nC2, nC3 till nCn { hold_ncr_values[r] = factorial(n) / (factorial(n-r) * factorial(r)); } } void computeBezierPoints(float t, point *actual_bezier_point, int number_of_control_points, point *control_points_array, int *hold_ncr_values) { int i, n = number_of_control_points-1; float bernstein_polynomial; actual_bezier_point -> x = 0; actual_bezier_point -> y = 0; actual_bezier_point -> z = 0; for(i=0; i < number_of_control_points; i++) { bernstein_polynomial = hold_ncr_values[i] * pow(t, i) * pow( 1-t, n-i); actual_bezier_point -> x += bernstein_polynomial * control_points_array[i].x; actual_bezier_point -> y += bernstein_polynomial * control_points_array[i].y; actual_bezier_point -> z += bernstein_polynomial * control_points_array[i].z; } } void bezier(point *control_points_array, int number_of_control_points, int number_of_bezier_points) { point actual_bezier_point; float t; int *hold_ncr_values, i; hold_ncr_values = new int[number_of_control_points]; // to hold the nCr values computeNcR(number_of_control_points-1, hold_ncr_values); // call nCr function to calculate nCr values glBegin(GL_LINE_STRIP); for(i=0; i<=number_of_bezier_points; i++) { t=float(i)/float(number_of_bezier_points); computeBezierPoints(t, &actual_bezier_point, number_of_control_points, control_points_array, hold_ncr_values); glVertex2f(actual_bezier_point.x, actual_bezier_point.y); } glEnd(); delete[] hold_ncr_values; } void display() { glClear(GL_COLOR_BUFFER_BIT); int number_of_control_points = 4, number_of_bezier_points = 20; point control_points_array[4] = {{100, 400, 0}, {150, 450, 0}, {250, 350, 0},{300, 400, 0}}; control_points_array[1].x += 50*sin(theta * PI/180.0); control_points_array[1].y += 25*sin(theta * PI/180.0); control_points_array[2].x -= 50*sin((theta+30) * PI/180.0); control_points_array[2].y -= 50*sin((theta+30) * PI/180.0); control_points_array[3].x -= 25*sin((theta-30) * PI/180.0); control_points_array[3].y += sin((theta-30) * PI/180.0); theta += 2; glPushMatrix(); glPointSize(5); glColor3f(1, 0.4, 0.2); //Indian flag: Saffron color code for(int i=0; i<50; i++) { glTranslatef(0, -0.8, 0 ); bezier(control_points_array, number_of_control_points, number_of_bezier_points); } glColor3f(1, 1, 1); //Indian flag: white color code for(int i=0; i<50; i++) { glTranslatef(0, -0.8, 0); bezier(control_points_array, number_of_control_points, number_of_bezier_points); } glColor3f(0, 1, 0); //Indian flag: green color code for(int i=0; i<50; i++) { glTranslatef(0, -0.8, 0); bezier(control_points_array, number_of_control_points, number_of_bezier_points); } glPopMatrix(); glLineWidth(5); glColor3f(0.7, 0.5,0.3); //pole colour glBegin(GL_LINES); glVertex2f(100,400); glVertex2f(100,40); glEnd(); glutPostRedisplay(); glutSwapBuffers(); } void init() { glMatrixMode(GL_PROJECTION); glLoadIdentity(); gluOrtho2D(0,500,0,500); } int main(int argc, char **argv) { glutInit(&argc, argv); glutInitDisplayMode(GLUT_DOUBLE | GLUT_RGB); glutInitWindowPosition(0, 0); glutInitWindowSize(500,500); glutCreateWindow("Bezier Curve - updated"); init(); glutDisplayFunc(display); glutMainLoop(); } |
Advertisement
INDIA FLAG
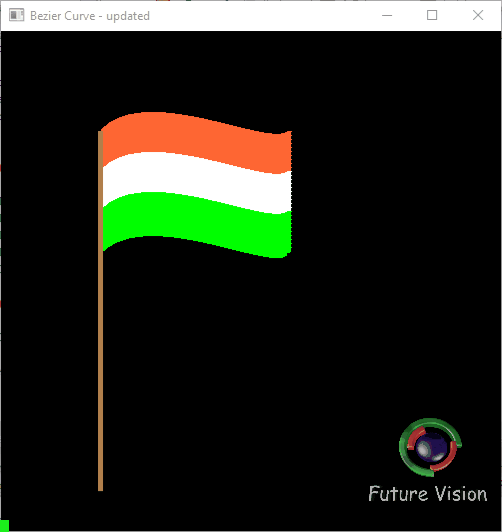
Fig 1.1: ANIMATED OUTPUT .
Advertisement
×
Note
Please Share the website link with Your Friends and known Students...
-ADMIN
-ADMIN
×
Note
Page Number is specified to navigate between Pages...
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
Advertisement