×
NOTE!
Click on MENU to Browse between Subjects...
Advertisement
COMPUTER GRAPHICS LABORATORY WITH MINI PROJECT
[As per Choice Based Credit System (CBCS) scheme]
(Effective from the academic year 2017 - 2018)
SEMESTER - VI
Subject Code 17CSL68
IA Marks 40
Number of Lecture Hours/Week 01I + 02P
Exam Marks 60
Advertisement
17CSL68 - COMPUTER GRAPHICS LABORATORY WITH MINI PROJECT
PROGRAM - 9
Develop a menu driven program to fill the polygon using scan line algorithm
Code Credits Prof Shankar R, BMSIT
DESIGN Credits Mr K B Hemanth Raj - Admin
Advertisement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 | // Scan-Line algorithm for filling a polygon #include <stdlib.h> #include <stdio.h> #include <GL/glut.h> float x1, x2, x3, x4, y1, y2, y3, y4; // our polygon has 4 lines - so 8 coordinates void edgedetect(float x1, float y1, float x2, float y2, int *left_edge, int *right_edge) { float x_slope, x, temp; int i; if ((y2-y1)<0) // decide where to start { temp = y1; y1 = y2; y2 = temp; temp = x1; x1 = x2; x2 = temp; } if ((y2-y1)!=0) x_slope = (x2 - x1) / (y2 - y1); else x_slope = x2 - x1; x = x1; for (i = y1; i <= y2; i++) { if (x < left_edge[i]) left_edge[i] = x; if (x > right_edge[i]) right_edge[i] = x; x = x + x_slope; } } void draw_pixel (int x, int y) { glColor3f (1, 1, 0); glBegin (GL_POINTS); glVertex2i (x, y); glEnd (); } void scanfill (float x1, float y1, float x2, float y2, float x3, float y3, float x4, float y4) { int left_edge[500], right_edge[500]; int i, y; for (i = 0; i <= 500; i++) { left_edge [i] = 500; right_edge [i] = 0; } edgedetect (x1, y1, x2, y2, left_edge, right_edge); edgedetect (x2, y2, x3, y3, left_edge, right_edge); edgedetect (x3, y3, x4, y4, left_edge, right_edge); edgedetect (x4, y4, x1, y1, left_edge, right_edge); for (y = 0; y <= 500; y++) { if (left_edge[y] <= right_edge[y]) { for (i = left_edge[y]; i <= right_edge[y]; i++) { draw_pixel (i, y); glFlush (); } } } } void display() { x1 = 200, y1 = 200; x2 = 100, y2 = 300; x3 = 200, y3 = 400; x4 = 300, y4 = 300; glClear (GL_COLOR_BUFFER_BIT); glColor3f (0, 0, 1); glBegin (GL_LINE_LOOP); glVertex2f (x1, y1); glVertex2f (x2, y2); glVertex2f (x3, y3); glVertex2f (x4, y4); glEnd (); scanfill (x1, y1, x2, y2, x3, y3, x4, y4); } void init() { glClearColor (1, 1, 1, 1); gluOrtho2D (0, 499, 0, 499); } int main (int argc, char** argv) { glutInit (&argc, argv); glutInitDisplayMode (GLUT_SINGLE|GLUT_RGB); glutInitWindowSize (500, 500); glutInitWindowPosition (0, 0); glutCreateWindow ("Filling a Polygon using Scan-line Algorithm"); init (); glutDisplayFunc (display); glutMainLoop (); } |
Advertisement
POLYGON IS FILLING COLOR (PIXEL BY PIXEL)
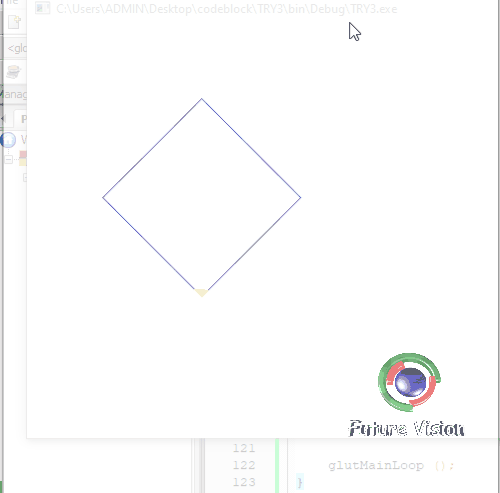
Fig 1.1: ANIMATED OUTPUT .
Advertisement
×
Note
Please Share the website link with Your Friends and known Students...
-ADMIN
-ADMIN
×
Note
Page Number is specified to navigate between Pages...
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
Advertisement