×
NOTE!
Click on MENU to Browse between Subjects...
Advertisement
17CSL57
COMPUTER NETWORK LABORATORY
[As per Choice Based Credit System (CBCS) scheme]
(Effective from the academic year 2017-2018)
SEMESTER - V
This Page Provides Program & Output.
Program 8
Program 8
Write a program to find the shortest path between vertices using bellman-ford
algorithm.
Advertisement
BELLMANFORD.java
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 | import java.util.Scanner; class BELLMANFORD { static int n, dest; static double[] prevDistanceVector, distanceVector; static double[][] adjacencyMatrix; public static void main(String[] args) { Scanner scanner = new Scanner(System.in); System.out.println("Enter number of nodes"); n = scanner.nextInt(); adjacencyMatrix = new double[n][n]; System.out.println("Enter Adjacency Matrix (Use 'Infinity' for No Link)"); for (int i = 0; i < n; i++) for (int j = 0; j < n; j++) adjacencyMatrix[i][j] = scanner.nextDouble(); System.out.println("Enter destination vertex"); dest = scanner.nextInt(); distanceVector = new double[n]; for (int i = 0; i < n; i++) distanceVector[i] = Double.POSITIVE_INFINITY; distanceVector[dest - 1] = 0; bellmanFordAlgorithm(); System.out.println("Distance Vector"); for (int i = 0; i < n; i++) { if (i == dest - 1) { continue; } System.out.println("Distance from " + (i + 1) + " is " + distanceVector[i]); } System.out.println(); } static void bellmanFordAlgorithm() { for (int i = 0; i < n - 1; i++) { prevDistanceVector = distanceVector.clone(); for (int j = 0; j < n; j++) { double min = Double.POSITIVE_INFINITY; for (int k = 0; k < n; k++) { if (min > adjacencyMatrix[j][k] + prevDistanceVector[k]) { min = adjacencyMatrix[j][k] + prevDistanceVector[k]; } } distanceVector[j] = min; } } } } |
Process to Execute the Program
Step 1: We need to have Java JDK installed, So That Java Programs can Run.
Step 2: Copy & Paste the Below Code of BELLMANFORD.java.
Step 3: or simple Download the Source Code.
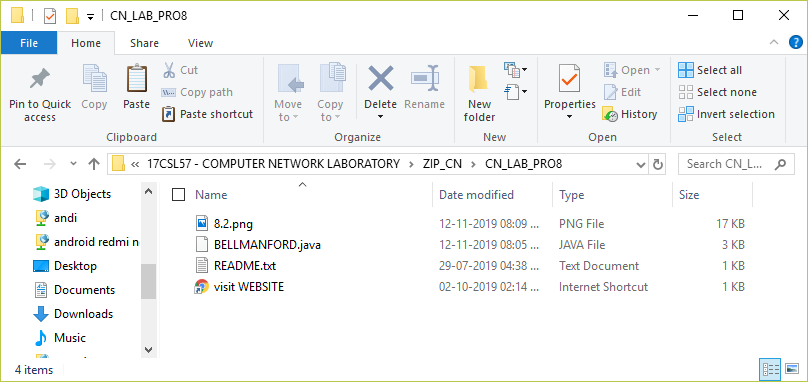
Fig 8.1: Required Files .
Step 5: Navigate to the BELLMANFORD.java File location.
Step 6: Use CD & DIR command on cmd to navigate.
Step 7: First Run the BELLMANFORD.java => javac BELLMANFORD.java
Step 8: => java BELLMANFORD
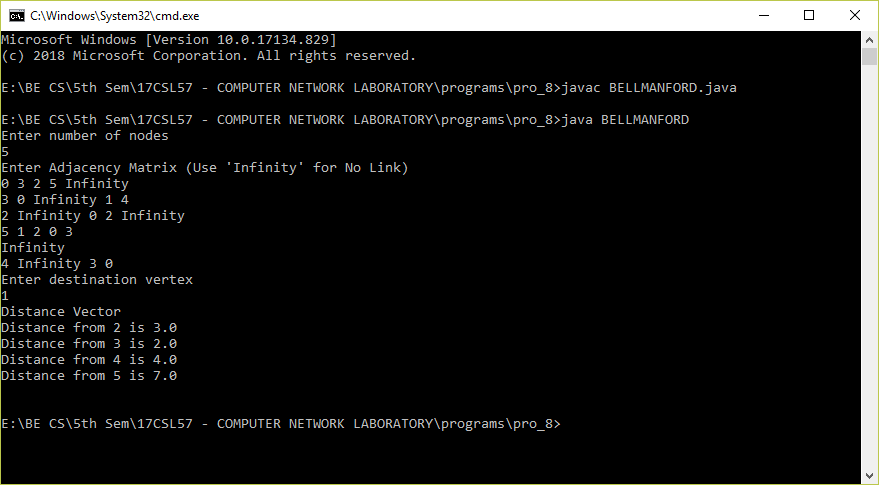
Fig 8.2: Demonstration of BELLMANFORD.java .
Step 10: Now Enter the Adjacency Matrix & Type "Infinity" in case distance is unknown.
Step 11: Enter the Destination Vertex
Step 12: Get the Shortest Distance from the Destination Vertex.
×
Note
Please Share the website link with Your Friends and known Students...
-ADMIN
-ADMIN
×
Note
Page Number is specified to navigate between Pages...
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN