×
NOTE!
Click on MENU to Browse between Subjects...
Advertisement
DESIGN AND ANALYSIS OF ALGORITHMS LABORATORY
(Effective from the academic year 2018 -2019)
SEMESTER - IV
Course Code 18CSL47
CIE Marks 40
Number of Contact Hours/Week 0:2:2
SEE Marks 60
Total Number of Lab Contact Hours 36
Exam Hours 03
Experiments 8
Find Minimum Cost Spanning Tree of a given connected undirected graph using Kruskal's Algorithm. Use Union-Find algorithms in your program.
Advertisement
Advertisement
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 | import java.util.Arrays; import java.util.Scanner; class Edge { int src; int dest; int weight; Edge(int src, int dest, int weight) { this.src = src; this.dest = dest; this.weight = weight; } } class Kruskal { public static void main(String[] args) { Scanner scan = new Scanner(System.in); System.out.println("Enter number of Vertices"); int n = scan.nextInt(); int adj[][] = new int[n][n]; System.out.println("Enter Adjacency Matrix"); for (int i = 0; i < n; i++) { for (int j = 0; j < n; j++) { adj[i][j] = scan.nextInt(); } } scan.close(); // Maximum Edges without any Loops can be ((n * (n - 1)) / 2). Edge[] edges = new Edge[(n * (n - 1)) / 2]; int k = 0; for (int i = 0; i < n; i++) { for (int j = i + 1; j < n; j++) { edges[k] = new Edge(i, j, adj[i][j]); k++; } } sort(edges); // Declare an array of size vertices to keep track of respective leaders of each element. int[] parent = new int[n]; // Assign each element of array of with value -1. Arrays.fill(parent, -1); int minCost = 0; System.out.println("Edges: "); for (int i = 0; i < k; i++) { // Find the super most of leader of source vertex. int lsrc = find(parent, edges[i].src); // Find the super most of leader of destination vertex. int ldest = find(parent, edges[i].dest); // If those two leaders are different then they belong to isolated groups. if (lsrc != ldest) { System.out.println((edges[i].src + 1) + " <-> " + (edges[i].dest + 1)); minCost += edges[i].weight; union(parent, lsrc, ldest); } } System.out.println(); System.out.println("Minimum Cost of Spanning Tree: " + minCost); } static void sort(Edge[] edges) { // Sort Edges according to their weights using Bubble Sort. for (int i = 1; i < edges.length; i++) { for (int j = 0; j < edges.length - i; j++) { if (edges[j].weight > edges[j + 1].weight) { Edge temp = edges[j]; edges[j] = edges[j + 1]; edges[j + 1] = temp; } } } } static int find(int[] parent, int i) { if (parent[i] == -1) { // Super Most Leader Element Found. return i; } // Find Above Leader in recurrsive manner. return find(parent, parent[i]); } static void union(int[] parent, int lsrc, int ldest) { // Make destination vertex leader of source vertex. parent[lsrc] = ldest; } } |
Advertisement
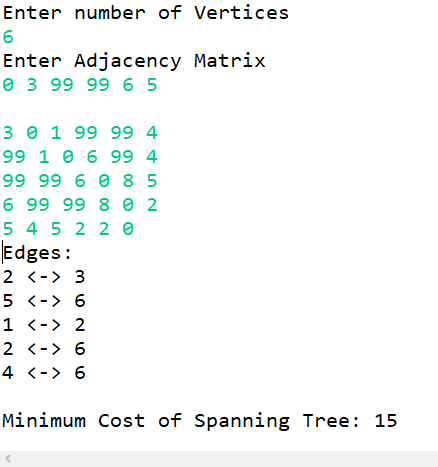
Fig 8: Output .
×
Note
Please Share the website link with Your Friends and known Students...
-ADMIN
-ADMIN
×
Note
Page Number is specified to navigate between Pages...
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
T = Text book
QB = Question Bank
AS = Amswer Script
-ADMIN
Advertisement